Creating and Reusing the Code Snippet in Xcode
Developers often run into situations where they need to use a similar snippet of code again and again. It's a lot of effort trying to find it in the codebase, from a random blog or the StackOverflow.
Xcode offers a way to save any code snippet and later retrieve it using a simple keyword. You can choose any keyword you want to associate with the saved snippet so that you can retrieve the whole code just with a few keystrokes.
We will understand the snippet saving feature by going through the following list of activities and see how it is done,
- Saving a snippet
- Loading a snippet
- Viewing and Editing snippets
- Importing and Exporting snippets from and to Xcode
tl;dr;
If you haven't got the time to go through the entire article, I made a video with major parts of the article that will give you a good understanding of how snippets creation and usage happens.
Saving a snippet
Let's say, you find yourself using the code snippet to artificially add a delay in your code. For example, I use it all the time when I need to mimick the web request behavior,
DispatchQueue.main.asyncAfter(deadline: .now() + 2.0) {
// Code you want to be delayed
}
After adding a code snippet, you can decide which values you want to be changeable once this snippet is included. For example, I want the delay to be editable. In order to do that I can replace the hardcoded value of 2
in the above example with the placeholder text and placeholder markers like this before saving it in the snippet library.
DispatchQueue.main.asyncAfter(deadline: .now() + <#Delay in seconds#>) {
// Code you want to be delayed
}
If you take a look at this code in Xcode, it will look like this.

Meaning, the developer can replace the value enclosed in <##>
markers with any delay value then want.
For example, if I want the input parameters of my code snippet function to be a placeholder, I can simply write it asdoMatch(<#placeholder#>)
. Now you can replaceplaceholder
with any value once the snippet is loaded in the Xcode
To save the snippet, select the piece of code you want to save, right-click and choose Create Code Snippet
option.
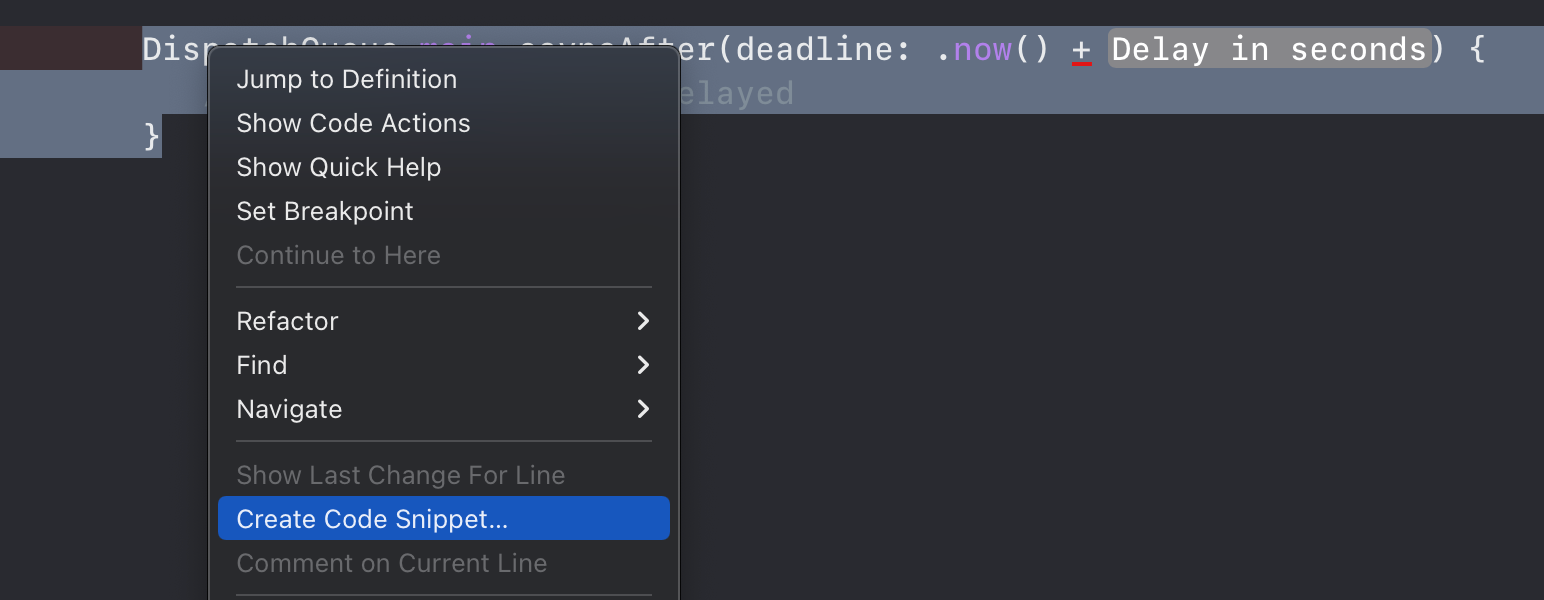
Next, it will show you a window asking you to fill all the parameters associated with the snippet completion.
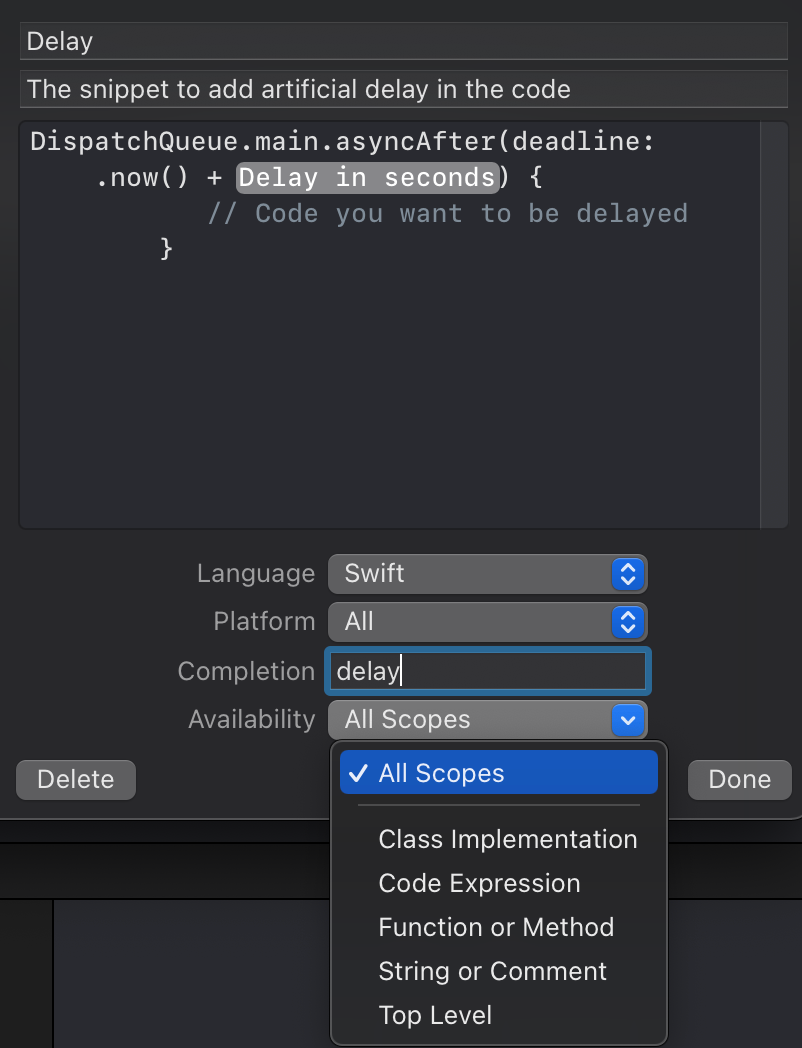
Below is the summary of all the parameters you can specify before saving,
- Title
- Summary
- The snippet (You can still make changes to it before saving)
- Supported languages
- Supported platforms
- The completion keyword. The keyword that will be used to import the snippet in your code
- Scope availability. Where do you want this snippet to be available? Possible options are
Class implementation
,code expression
,function
ormethod
, and so on
Once you fill in all the details, press done
and voilà! Your snippet is ready to use.
Loading a snippet
Once you save the snippet, loading it is just a few keystrokes away. For example, I saved the previous piece of code with the completion keyword as delay
and I can start typing delay
. While I am doing that, Xcode will give me the completion option.
Since I left a placeholder text with Delay in seconds
, I can replace it with any value I want and this applies to any snippet you create with any number of placeholder parameters.
Viewing and Editing snippets
To view all the snippets, you can go Xcode -> View -> Show Library
where you can see all your snippets plus those that came in with Swift.
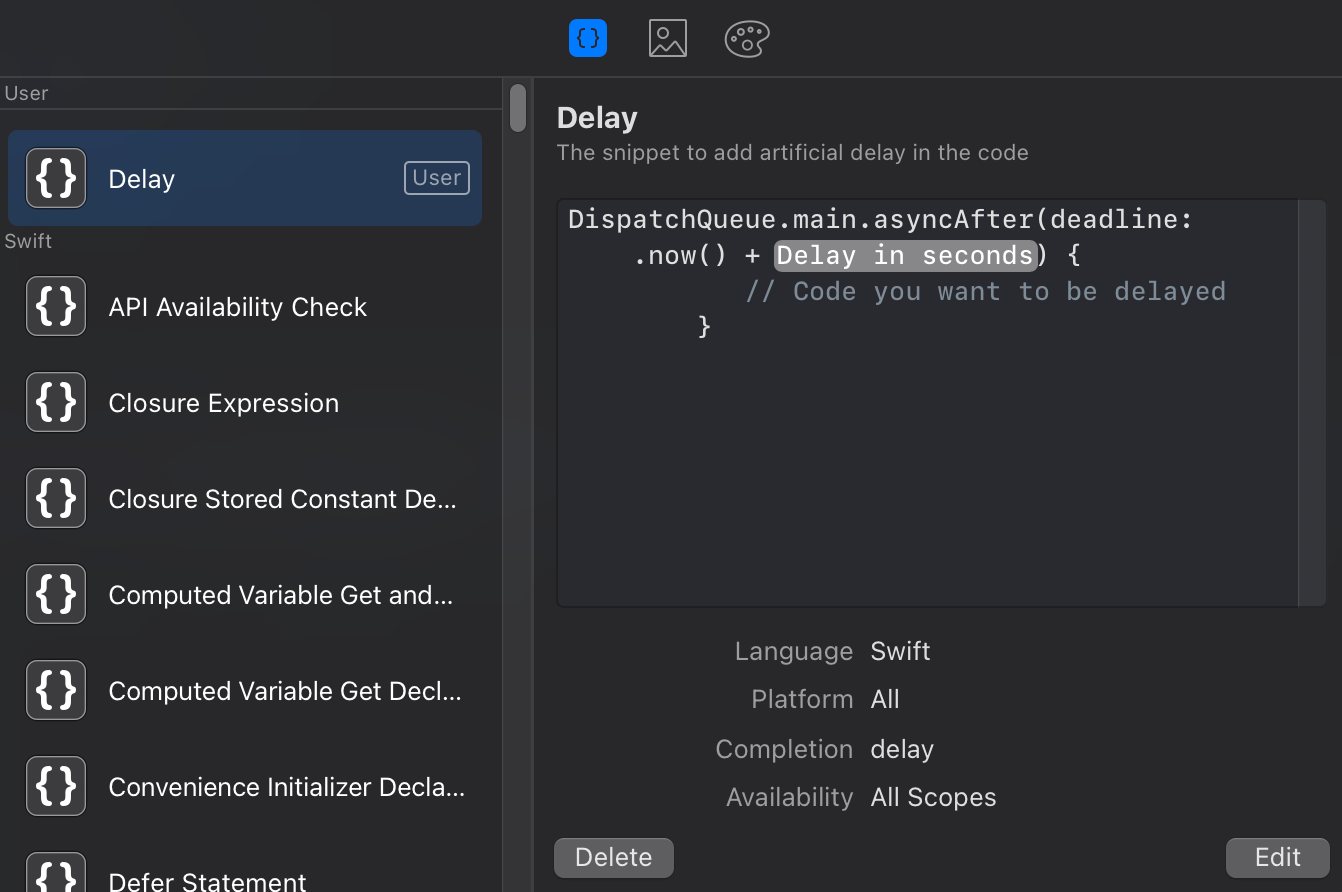
To edit the code snippet, select and press the edit button. Make any changes you want and press the Done
button. The edited snippet is ready for use.
Importing and Exporting snippets from and to Xcode
Often you will run into situations where you might want to import and export snippets between different Xcode versions or different machines. Xcode offers an easy way to do this.
To import all the snippets, navigate to this directory by running this command from the terminal,
cd ~/Library/Developer/Xcode/UserData/CodeSnippets
This was the default location for all the Xcode snippets as of Xcode Version 13.0 beta 4. This may change in the future, but for now, it suffices to use this path to load snippets
In this path, you can find the xml
file with the name in the following format
<long_identifier>.codesnippet
This file represents the single snippet in your Xcode. If you have multiple code snippets, you will find multiple xml
files in this location. To export it to another machine where you want to reuse it, simply copy and paste this file to that location.
Once you move the snippet files, make sure to restart the Xcode to be able to detect and track these snippet files.
Bonus Content
You can still manually edit the snippets by directly editing these xml
files. For example, the xml
file for my recent snippet looks something like this,
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>IDECodeSnippetCompletionPrefix</key>
<string>delay</string>
<key>IDECodeSnippetCompletionScopes</key>
<array>
<string>All</string>
</array>
<key>IDECodeSnippetContents</key>
<string>DispatchQueue.main.asyncAfter(deadline: .now() + <#Delay in seconds#>) {
// Code you want to be delayed with few seconds
}</string>
<key>IDECodeSnippetIdentifier</key>
<string>66308436-700D-40D2-82B1-C5CBC337A731</string>
<key>IDECodeSnippetLanguage</key>
<string>Xcode.SourceCodeLanguage.Swift</string>
<key>IDECodeSnippetSummary</key>
<string>The snippet to add artificial delay in the code</string>
<key>IDECodeSnippetTitle</key>
<string>Custom Delay</string>
<key>IDECodeSnippetUserSnippet</key>
<true/>
<key>IDECodeSnippetVersion</key>
<integer>2</integer>
</dict>
</plist>
I can open this snippet in the editor and edit it in the same way I do it in Xcode and save it. Unfortunately, snippet changes done this way are not immediately caught by Xcode. So, please make sure to restart the Xcode before you see changes done in the xml
file getting reflected in the Xcode.
WWDC Presenters Often Use the Code Snippets
Presenting at WWDC is nerve-wracking, especially when the eyes of all iOS developers are on you. A lot of things can go wrong and you don't want to risk it by typing wrong and non-buildable code. To simplify things, you will see Apple developers often storing part of their code using snippets and retrieving it using just a few keystrokes.
Summary
I hope you got a good idea about how to create and reuse snippets in Xcode. Snippets are a powerful tool to reuse existing functionality with a single-stop shop to find all the beautiful snippets you created for reuse.
If you liked the article, feel free to share it. If there is anything missing or needs improvement, feel free to message me on Twitter. Thanks for reading and until next time with another article.