Detecting internet connection status in JavaScript
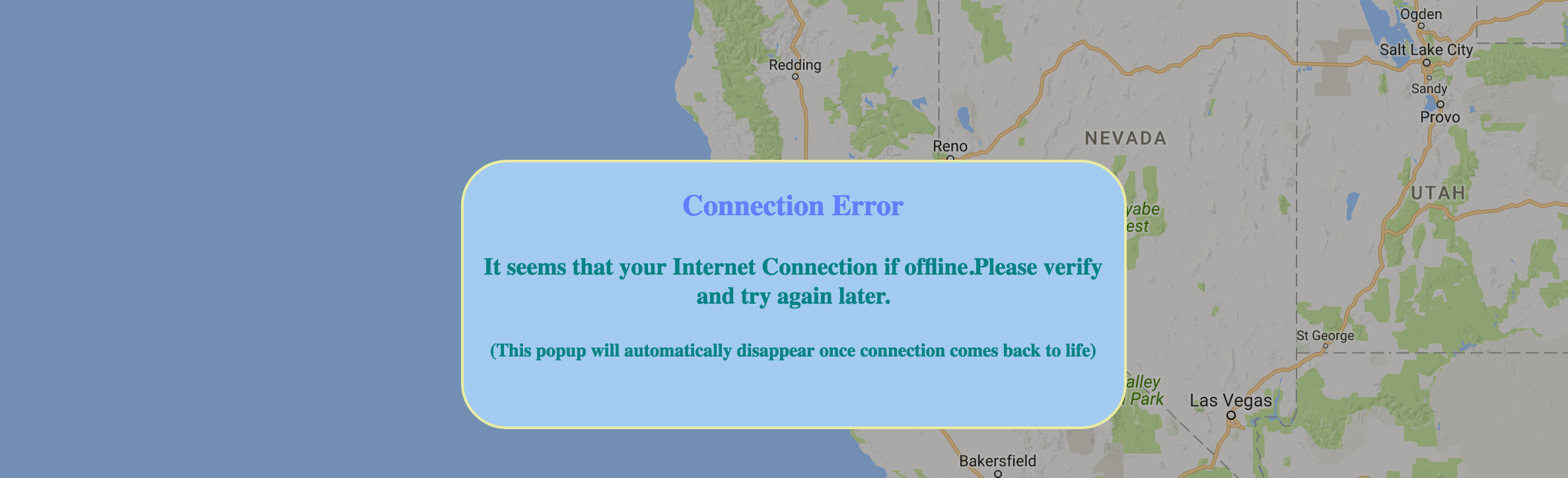
Detecting status of an internet connection in the web project could be crucial in terms of user experience. An app can take additional actions to cope up with lost connection or even alert user to check the internet settings or seek an alternate connection supply.
Detecting internet loss is very easy in JavaScript.
To quote the technical details of this API from W3schools.com
Return Value: A Boolean, indicating whether the browser is in online or offline mode.
Returns true if the browser is online, otherwise it returns false
You can read more about this property on Mozilla Developer Network blog. Simple usage example as taken from the MDN itself
var isOnline = window.navigator.onLine;
if (isOnline) {
console.log('online');
} else {
console.log('offline');
}
However, this is inconvenient since it gives status only once per load. But what happens if network is available when page was loaded but they fluctuates later on. How do you detect connection loss/recovery in this case?
Yes, you can detect the internet loss dynamically by adding online/offline
event listeners as follows,
// Add event listener offline to detect network loss.
window.addEventListener("offline", function(e) {
showPopForOfflineConnection();
});
// Add event listener online to detect network recovery.
window.addEventListener("online", function(e) {
hidePopAfterOnlineInternetConnection();
});
function hidePopAfterOnlineInternetConnection(){
// Enable to text field input
$("#input-movie-name").prop('disabled', false);
// Enable the search button responsible for triggering the search activity
$("#search-button").prop('disabled', false);
// Hide the internet connection status pop up. This is shown only when connection if offline and hides itself after recovery.
$('#internet-connection-status-dialogue').trigger('close');
}
function showPopForOfflineConnection(){
// Disable the text field input
$("#input-movie-name").prop('disabled', true);
// Disable the search button responsible for triggering search activity.
$("#search-button").prop('disabled', true);
// Show the error with appropriate message title and description.
$(".main-error-message").html("Connection Error");
$(".main-error-resolution").html(" It seems that your Internet Connection if offline.Please verify and try again later.");
$(".extra-error-message").html("(This popup will automatically disappear once connection comes back to life)");
// Addition of extra design to improve user experience when connection goes off.
$('#internet-connection-status-dialogue').lightbox_me({
centered: true,
overlaySpeed:"slow",
closeClick:false,
onLoad: function() {
}
});
}
As pointed out by MDN, it is supported on all modern desktop browsers so you don't have to worry about compatibility issue