How to Initialize State and StateObject in SwiftUI
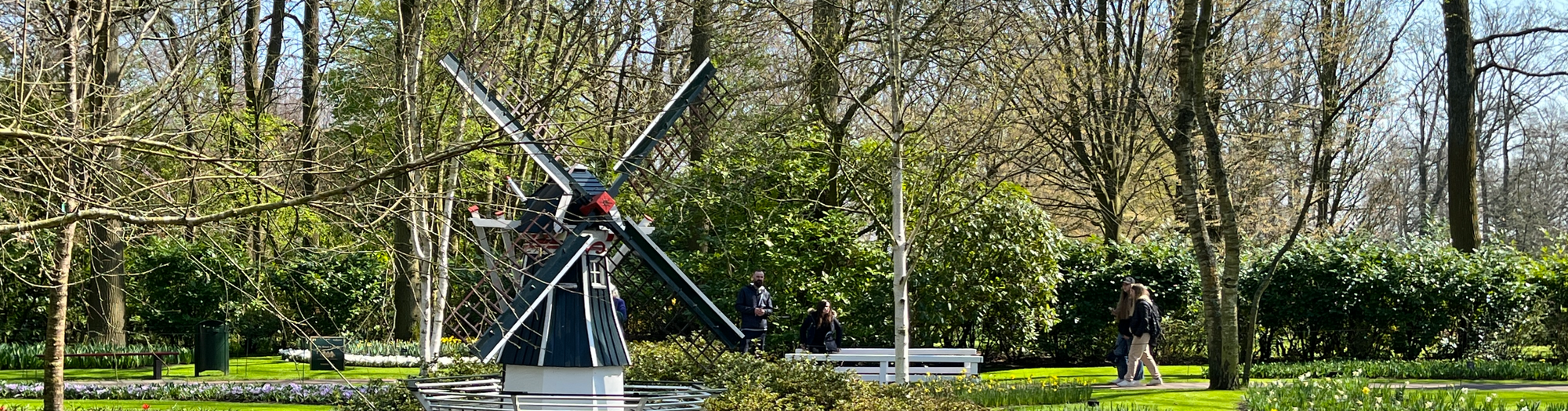
State
and StateObject
are standard mechanisms in SwiftUI to maintain and update the view state. However, their initialization is not intuitive. Unlike regular Swift variables, you can directly instantiate them. They can only be initialized in certain ways while using them in the Swift code.
In today's blog post, we will see how to initialize State and StateObject correctly in SwiftUI.
How to Correctly Initialize State Variable in SwiftUI
State variables are often used to modify and signal changes in values that control the UI. Below, you will see how to correctly initialize the state variable isLoading
in StateExampleView
view,
struct StateExampleView {
@State private var isLoading: Bool
init(isLoading: Bool) {
_isLoading = State(initialValue: isLoading)
}
}
isLoading
is declared a private variable. State variables are used only within the containing view so are not meant to be used outside. If you're using them outside of the containing view, there is something wrong with your architectureHow to Correctly Initialize StateObject Variable in SwiftUI
State objects are used to modify and signal changes in multiple values (Which are part of the same object) that control the UI. Below, you will see how to correctly initialize the state variable viewState
in StateExampleView
view.
State objects being the observed properties need to conform to ObservableObject
protocol and should be classes. Any observed properties on state objects are declared with @Published
annotation.
final class ViewState: ObservableObject {
@Published var title: String
@Published var subtitle: String
@Published var isLoading: Bool
init(title: String, subtitle: String, isLoading: Bool) {
self.title = title
self.subtitle = subtitle
self.isLoading = isLoading
}
}
struct StateExampleView {
@StateObject private var viewState: ViewState
init(isLoading: Bool) {
_viewState = StateObject(wrappedValue: ViewState(title: "No Title", subtitle: "No Subtitle", isLoading: false))
}
}
Summary
That's all folks for today's short blog article. Hope it gave you a good overview of how to properly initialize state properties on SwiftUI
view. If you have any questions, comments, concerns, or follow-ups, please reach out to me on LinkedIn.