How to Perform Custom Action on a load of SwiftUI view
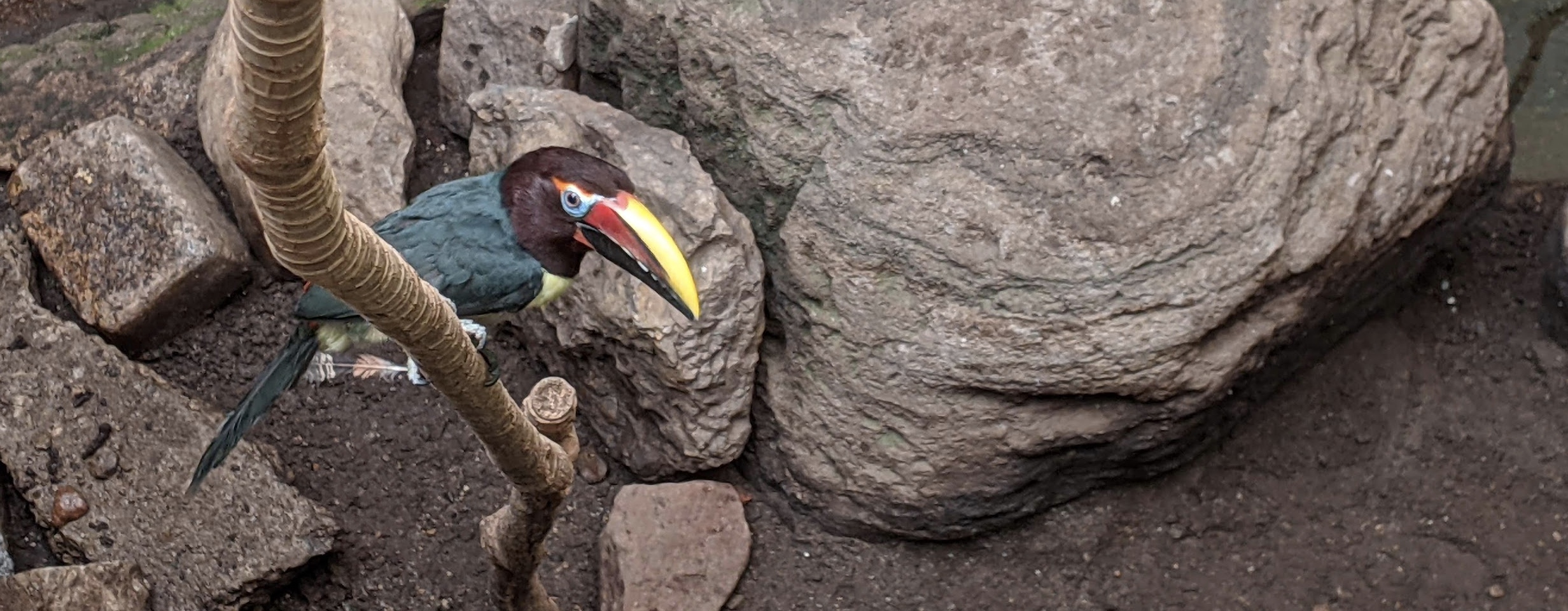
UIKit
offers a convenient viewDidLoad
method that gets executed as soon as the ViewController
is loaded and is called just once in the view controller lifecycle.
Unfortunately, SwiftUI
has no such feature. There is no way to perform the action just once after the view is loaded. Today, we are going to learn how to write our own viewDidLoad
method for SwiftUI
views that executes a given piece of code just once after the view has been loaded.
Let's go step-by-step through creating a solution,
- Building a
ViewModifier
The first step in building our custom viewDidLoad
method is creating a ViewModifier
. As SwiftUI
dictates, it is going to conform to ViewModifier
protocol. We will pass the closure in its initialization which will get called as soon as the view appears for the first time.
In order to keep track of the state and avoid repeat execution, we use the State
variable didViewLoad
, which gets set to true
after the first call. Future calls are ignored since we execute the closure only when didViewLoad
flag is false.
struct CustomViewDidLoadModifier: ViewModifier {
@State private var didViewLoad = false
private let action: (() -> Void)?
init(perform action: (() -> Void)? = nil) {
self.action = action
}
func body(content: Content) -> some View {
content.onAppear {
if !didViewLoad {
didViewLoad = true
action?()
}
}
}
}
2. Convenience Method
To make using this view modifier easier, let's wrap it in a nice extension. We will add onLoad
method on the View
extension which will take the closure as the parameter. This closure will get executed just once after the view appears for the first time.
extension View {
func onLoad(perform action: (() -> Void)? = nil) -> some View {
modifier(CustomViewDidLoadModifier(perform: action))
}
}
3. Demo Time
Now that all parts are ready, let's integrate and see how the final code works.
We will try to load a custom CustomViewDidLoad
. We will call onLoad
method on it (As provided by the extension above) when it appears for the first time.
//CustomViewDidLoad.swift
struct CustomViewDidLoad: View {
var body: some View {
VStack {
Text("Hello")
}
}
}
//Demo
CustomViewDidLoad().onLoad {
print("YES")
}
If you run the app now, you can see YES
getting printed just once unless we go back and land on the CustomViewDidLoad
view screen again.
Summary
That's it for today's blog post. Hope you liked it. The technique mentioned in this post can be used to create any kind of view modifier and apply a transformation to your view. You could be applying any style, or capturing any action as demonstrated here. Using the same technique, you can also apply custom behavior like ignoring the first load and/or capturing the 2nd, 3rd, or nth load of the view. The possibilities are endless.
Source Code
The full source code for this tutorial is available on GitHub Gist for further reference
Support and Feedback
If you have any comments or questions, please feel free to reach out to me on LinkedIn.
If you like my blog content and wish to keep me going, please consider donating on Buy Me a Coffee or Patreon. Help, in any form or amount, is highly appreciated and it's a big motivation to keep me writing more articles like this.
Consulting Services
I also provide a few consulting services on Topmate.io, and you can reach out to me there too. These services include,