Generating random string in PHP
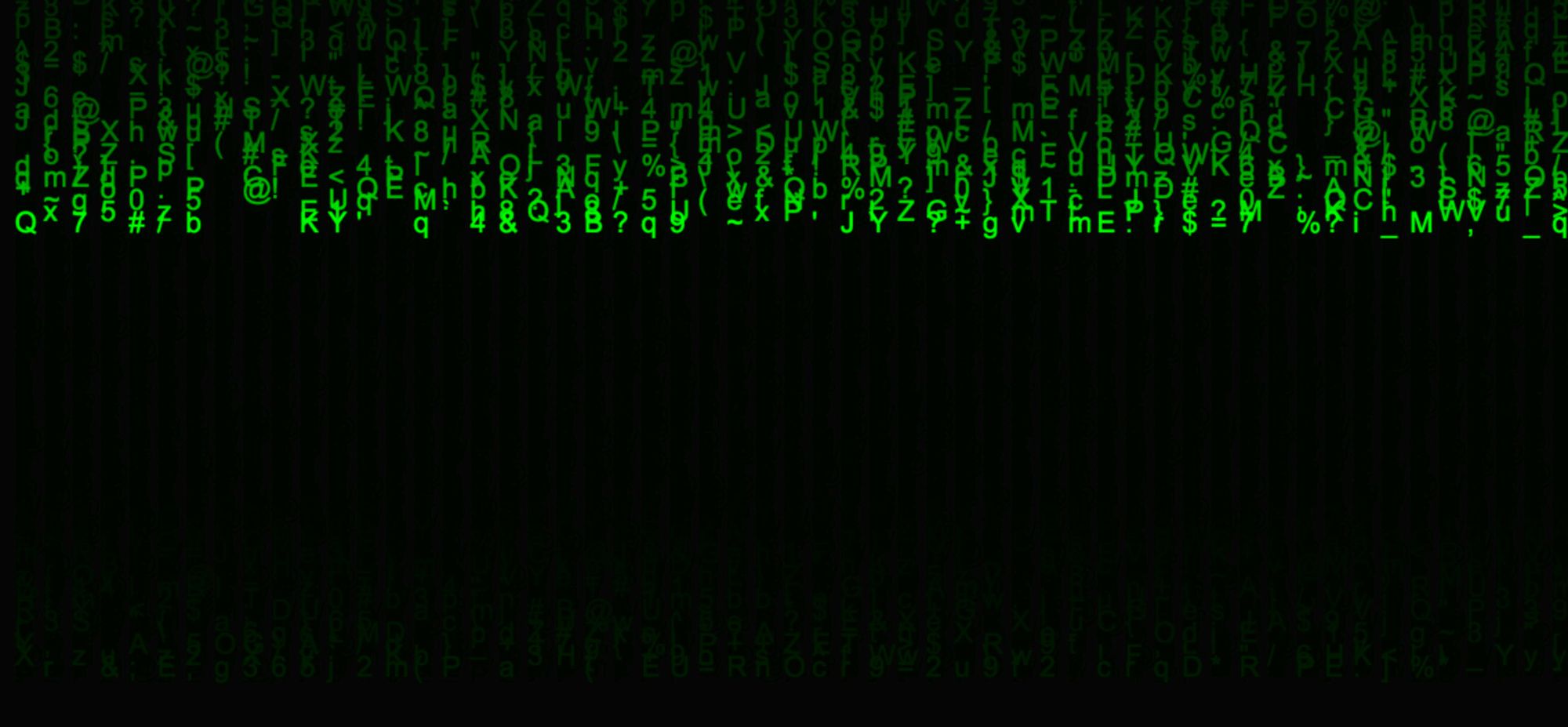
Many times using PHP
we developers require to generate a random string for obfuscation purpose. There could be lot of use-cases where this is applicable. Today, I will show you how to generate a random string of arbitrary length from the given character set.
For the sake of simplicity, let's assume that we have to generate a random string out of characters from following set.
$character_set = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789abcdefghijklmnopqrstuvwxyz_";
Remember that value of character set depends on the desired complexity. Update the character set if you want string with more/less security.
I would enlist a function which actually generates a random string based on the given character set.
function randString($length, $character_set) {
$str = '';
$count = strlen($character_set);
while ($length--) {
$str .= $character_set[mt_rand(0, $count-1)];
}
return $str;
}
// Then you can generate the random string based on the given character set by calling following method
$random_string = randString(10, $character_set);